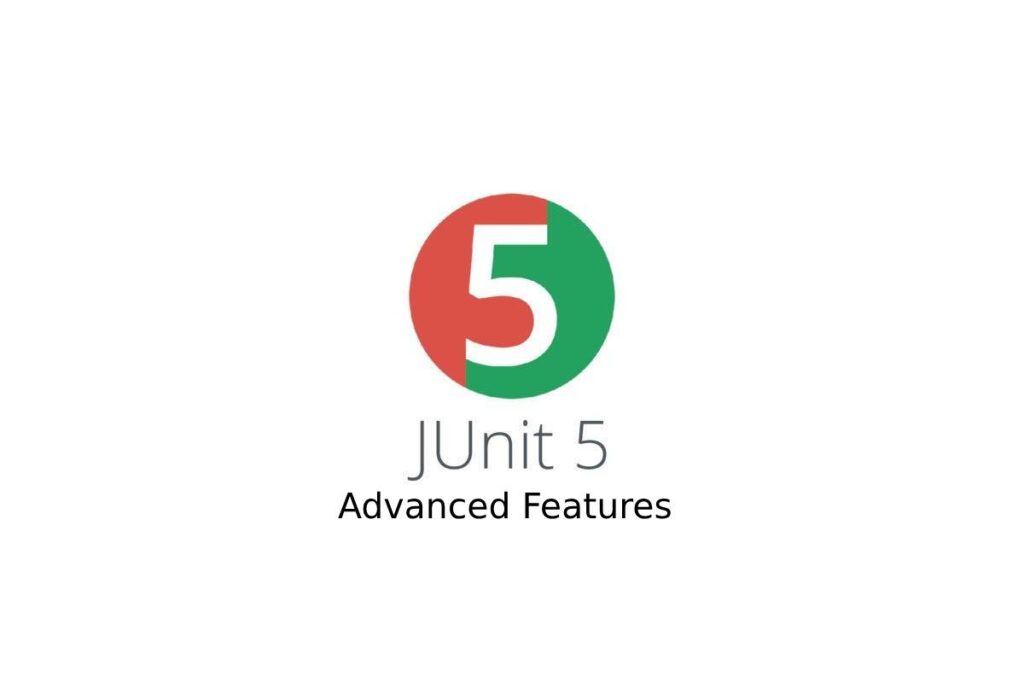
Testing has always been at the core of building reliable software, but as applications grow more complex, so do the challenges of ensuring their reliability. Developers and testers must balance speed with thoroughness, making tools like JUnit invaluable. JUnit testing stands out not only for its simplicity but also for the advanced capabilities it brings to modern testing practices.
By leveraging features like parameterized tests, extensions, and retries, you can enhance your test suite to handle complex scenarios with precision. These features aren’t just add-ons; they are practical tools that make testing more efficient and adaptable to the fast-paced demands of development cycles. Whether it’s streamlining validation for edge cases or addressing flaky tests, mastering JUnit testing is a step toward creating stronger, more resilient software.
Understanding the Importance of Advanced JUnit Features
You’re probably familiar with basic JUnit testing and functionalities that facilitate straightforward unit testing. However, as applications grow in complexity, relying solely on basic tests might not be enough. Advanced features enable you to write more flexible and powerful tests tailored to different scenarios. This isn’t just about making your life easier; comprehensive tests can lead to more reliable and maintainable code.
The landscape of software development continuously evolves, and tools like JUnit evolve, too, integrating advanced capabilities that increase the depth of your testing. With current discussions around Continuous Integration and Deployment (CI/CD) practices, enhancing your unit testing process is essential for keeping up with modern development cycles.
Parameterized Tests
Parameterized tests allow you to run the same test multiple times with different inputs. In real applications, this feature is useful to validate that a unit works correctly with a variety of data sets. Instead of multiple test cases for the different scenarios, you may have one common test case parameterized.
Imagine you’re testing a calculator class that has a method for adding two numbers. Instead of creating individual tests for every possible pair of numbers, a parameterized test lets you define a set of inputs—say, pairs of integers—and assert that your method returns the expected result for each pair.
Setting Up Parameterized Tests
Using JUnit 5, you can create a parameterized test using the @ParameterizedTest annotation. You can specify the input parameters through various sources, such as @ValueSource, @CsvSource, or even custom methods returning Stream<Arguments>.
Real-World Example
If you run a banking application, you’re likely dealing with multiple transaction scenarios like deposits, withdrawals, and transfers. You might want to test the Transaction class that accepts different amounts, transaction types, and fees. A parameterized test will let you cover all these combinations without duplicating code.
For instance, you can use @CsvSource to have various scenarios for your transaction test:
@ParameterizedTest
@CsvSource({
“deposit, 100, 100”,
“withdraw, 50, 50”,
“transfer, 200, 200”
})
void testTransaction(String type, int amount, int expected) {
Transaction transaction = new Transaction(type, amount);
assertEquals(expected, transaction.process());
}
By using parameterized tests effectively, code maintenance becomes easier, and it helps ensure your application behaves correctly under various conditions.
Extensions for Customization
JUnit 5 introduces an extension model that allows the extension of the test execution vocabulary and customization. It lets you add other functionalities such as logging, resource management, or error handling to it without cluttering your test classes. You can think of extensions as plugins for JUnit.
Use Case for Extensions
Consider a situation where your tests require setting up a database connection before execution and closing it afterward. Instead of repeating the connection logic in every test class, you can define a reusable extension.
class DatabaseExtension implements BeforeEachCallback, AfterEachCallback {
@Override
public void beforeEach(ExtensionContext context) {
// Set up database connection
}
@Override
public void afterEach(ExtensionContext context) {
// Close database connection
}
}
This reduces boilerplate code and promotes clean test design. You can also implement features like a custom assertion library through extensions, vastly improving the expressiveness of your tests.
Leverage LambdaTest with Extensions
As systems become more integrated, tools like LambdaTest come into play. LambdaTest is an AI-powered test orchestration and execution platform that lets you run manual and automated tests at scale with over 3000+ web browsers online, real devices, and OS combinations.
Using JUnit 5’s Extensions, you can integrate LambdaTest into your test workflows with minimal setup. For instance, you can create a custom extension that automates the configuration of LambdaTest’s environment, allowing your tests to run on different browser combinations without manual intervention.
Parameterized tests in JUnit 5 are ideal for testing web applications under different browser and device conditions. LambdaTest’s integration enables you to define parameterized tests that run seamlessly across multiple environments. For instance, you can use @CsvSource to test your application on Chrome, Firefox, and Safari in different operating systems.
Test Retries: Handling Flaky Tests
Flaky testing has been a problem we might never be able to solve given there are so many dependencies.
Why Do Flaky Tests Happen?
In the realm of software testing, flaky tests are those that sometimes pass and other times fail without any changes made to the codebase. These can be caused by external factors such as network issues, timing problems, or dependencies on external systems. Typically, flaky tests introduce frustration in CI/CD pipelines because they undermine the reliability of your test results.
Implementing Test Retries
JUnit 5 provides an efficient way to address flaky tests through its retry feature. With the @Retry annotation, you can specify how many times a test should rerun on failure before it is finally marked as failed. This feature is especially useful when you know that certain tests may fail intermittently due to environmental factors outside your control.
Real-World Scenario for Retries
Consider a scenario where you’re testing an API call that should return results but occasionally encounters network issues. Employing the retry mechanism helps in cases where a transient failure may skew your test results.
@Retry(value = 3)
@Test
void testApiCall() {
// Your API call goes here
assertEquals(“Expected result”, apiCall());
}
This simple addition enhances resilience, significantly improving the reliability of your test suite.
The Importance of Efficiency
As organizations embrace Agile methodologies, the need for efficient testing practices has surged. By utilizing the advanced features of JUnit 5, you can save time while increasing coverage, ensuring that you catch defects early on in the development process.
For instance, through parameterized tests, you can validate multiple inputs effectively, while extensions can give your tests more flexibility and scalability. Additionally, by managing flaky tests through automatic retries, you enhance your test suite’s stability.
Current Trends and JUnit 5
As of late 2023, the momentum behind JUnit has only grown stronger. More developers are adopting JUnit platforms due to their compatibility with modern CI/CD practices. The educational landscape is also shifting; training platforms are increasingly including JUnit best practices in their curriculum to keep pace with industry demands.
Additionally, as more organizations move to cloud infrastructure, tools like LambdaTest are becoming vital. Web applications are now tested rigorously across various devices and browsers, and JUnit’s advanced features make it easier to integrate such practices into existing workflows.
Best Practices for Leveraging Advanced JUnit 5 Features
While JUnit 5 offers powerful tools to enhance your testing process, their true potential lies in how effectively you use them. Below are some best practices to ensure you’re making the most of parameterized tests, extensions, and retries:
Keep Tests Modular and Readable
- Write tests that are easy to understand and maintain. Avoid cramming multiple assertions or complex logic into a single test. This is especially important with parameterized tests, where clarity in input-output relationships ensures your test cases are comprehensible.
- Use descriptive test method names that clearly indicate the purpose of the test, such as shouldCalculateTotalForMultipleItems(), instead of generic names like testTotalCalculation().
Organize Parameterized Test Data
- Use sources like @CsvSource or custom methods with @MethodSource to manage test data efficiently. For larger datasets, consider external files with @CsvFileSource. This keeps your test logic clean and separates data concerns from code.
- Validate your data sources to ensure they’re aligned with the test logic. Incorrect or poorly structured data can lead to false positives or debugging nightmares.
Leverage Extensions for Consistency
- Use extensions to handle repetitive setup and teardown logic, such as database connections, test logging, or environment configuration. This promotes reusability and keeps your test methods focused on the actual functionality being tested.
- Modularize extensions to ensure they can be reused across different test classes. For example, create a DatabaseExtension for database tests and a BrowserExtension for web testing scenarios.
Handle Flaky Tests Wisely
- Don’t rely solely on retries to fix flaky tests. Investigate and resolve root causes like poorly written assertions, timing issues, or external dependencies.
- Use the retry feature strategically, for instance, with tests that involve network calls or external APIs prone to intermittent failures. Document why a retry is necessary to avoid masking potential issues in the codebase.
Integrate with CI/CD Pipelines
- Ensure your advanced test configurations, like extensions or retries, are seamlessly integrated into your CI/CD pipelines. Parameterized tests should be designed to run efficiently in parallel, minimizing execution time without compromising coverage.
- Incorporate tools like LambdaTest to automate cross-browser and device testing within your CI/CD pipeline, using JUnit extensions to simplify setup and streamline integration.
Monitor and Optimize Performance
- Continuously evaluate the performance of your test suite. Parameterized tests with extensive datasets or retry logic can increase execution time, which might bottleneck your development pipeline.
- Use JUnit’s tagging feature with @Tag to group and run only relevant tests in specific scenarios, such as running quick sanity checks for pull requests and comprehensive tests for releases.
Adopt a Fail-Fast Mindset
- Configure your test suite to fail early if a critical issue arises. Use assertions and setup logic that quickly identifies and reports failures rather than allowing unnecessary test execution.
By following these best practices, you can make your test suite not only powerful but also maintainable and efficient. Advanced features in JUnit 5 are tools to simplify complexity—not add to it—so using them thoughtfully is key to achieving a robust testing strategy.
Conclusion
In summary, JUnit 5 has brought a plethora of advanced features that enable you to enhance your JUnit testing processes. Whether it’s through parameterized tests that streamline your validation efforts, extensions that allow custom setups, or retry mechanisms that manage flaky tests, there’s a wealth of functionality at your fingertips.
In the fast-paced world of software development, embracing these advanced features will not only ease the testing burden but also pave the way for building resilient applications. By leveraging tools like LambdaTest, you can make your testing strategy even more robust, enabling you to deploy software confidently and efficiently.
By focusing on these aspects, you not only improve the quality of your software but also align your practices with the ever-evolving technological landscape. As the industry shifts forward, keeping these advanced JUnit capabilities in your toolkit will be crucial for staying competitive and effective in your testing strategies.